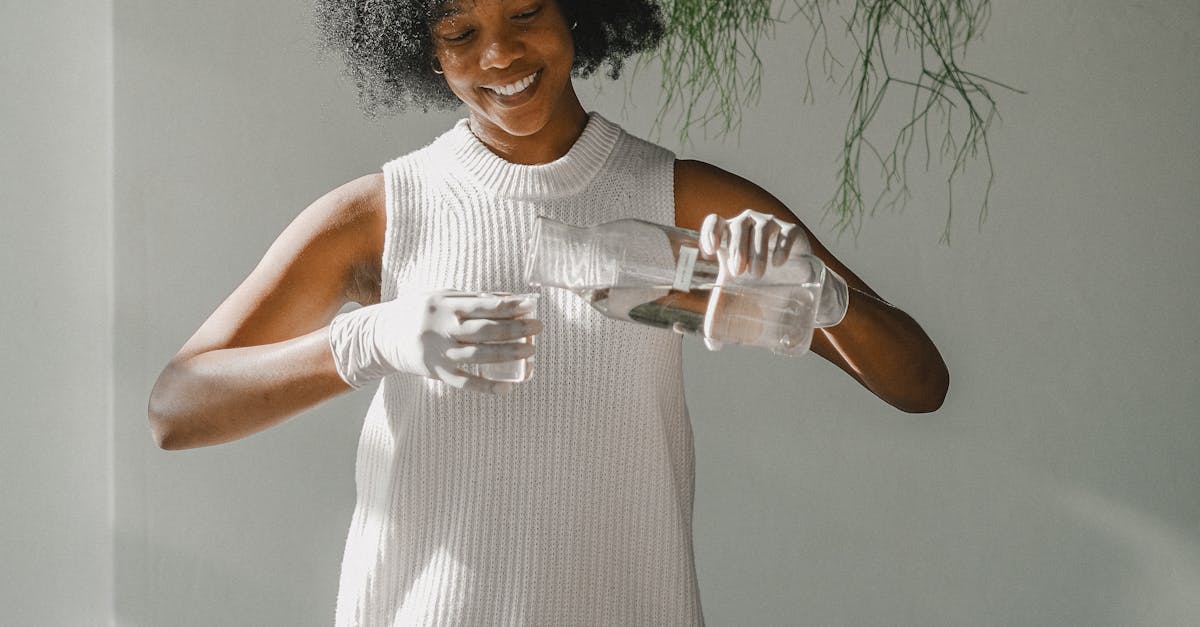
How to add matrices in c++?
There are two main ways to add matrices in C++: direct addition and addition using a summing vector. In direct addition, the matrices are added as if they were arrays, using a comma to separate the elements. For example, the addition of two matrices A and B, and C is given by A+B+C. Each element of the resulting matrix is the sum of the respective elements in the first two matrices. The addition of two vectors is similar: A+
How to add a vector to matrix in c++?
Adding a vector to a matrix can be done using the normal addition, addition assignment, or the += operator. The addition assignment is the same as adding to a column-wise vector, while the += adds to each row. However, if you are adding several vectors to a single matrix, you should use the addition assignment, otherwise you will end up adding each new vector to the previous result.
How to add elements to matrix in c++?
In c++, there are two main ways to add elements to a matrix. The first, using a function, adds all its elements at the same time. A character type or an int type can be added using the function called ‘operator+=’. The second method adds the elements one by one. The function ‘operator+=’ is used to add an element to the end of each row.
How to add a pointer to matrix in c++?
Pointers are variables that store the memory address of another variable. In c++, you can add pointers to an existing variable of another type to it. This is done by using the arrow (->) operator. The syntax is similar to adding an int to a pointer, except the arrow is used instead. In the code below, we declare a CMatrix variable and an int variable called x. The arrow operator is then used to add the CMatrix pointer to the int variable called x.
How to add an array to matrix in c++?
If you want to add an array to a matrix in c++, you need to use the function “insert”. The function “insert” allows you to add an element at a particular index and return the resulting matrix.