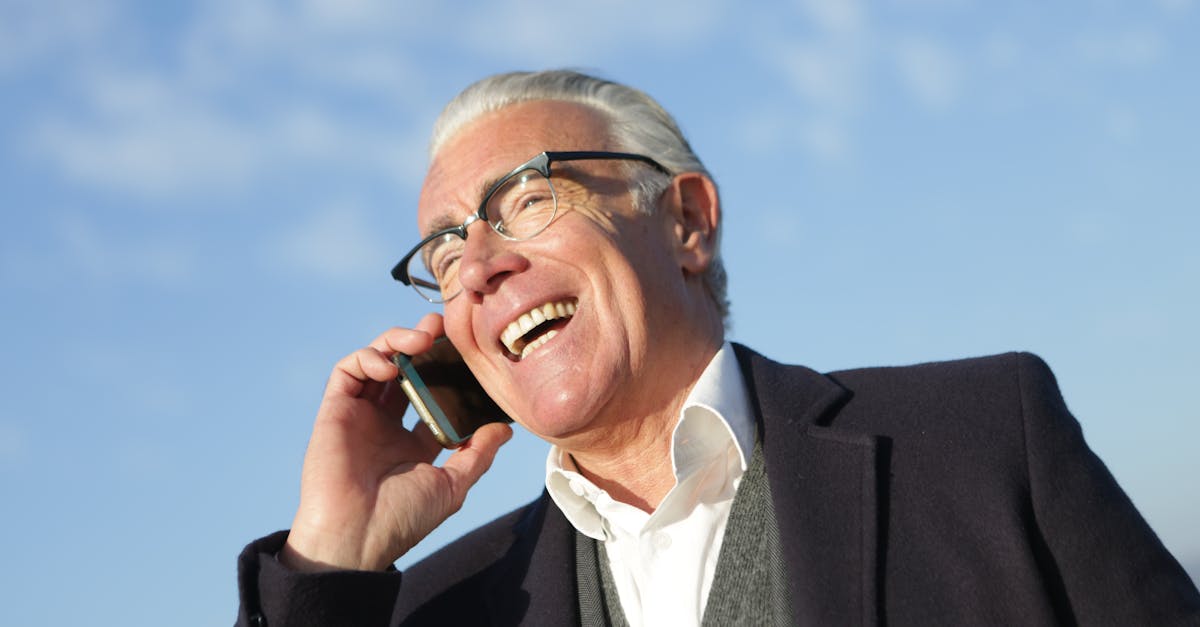
How to call a private method in java from another class?
Normally private methods are called using the keyword private which is prepended to the method name. However, it is possible to call the private methods of a class from another class. Using the keyword public before the method name will make it possible for other classes to call the method. This is known as access modifiers. Access modifiers are used to restrict the visibility of and access to the method to its class.
How to call a private method from another class in java?
If you want to call a private method from another class, you have two options: either create a public method that does the work of the private one or make the method protected. The first approach is the simplest. Just declare a method with the same name and a different access modifier (e.g. public void call privatemethod ) { privateMethod(); }) in the class where you want to call the method.
How to call a private method in java from another class without instantiating?
Use Accessor methods - Accessor methods are created to access the private and protected members of the class which are not accessible directly. An Accessor method is created by using the keyword private before the method declaration. The default keyword is public which allows the method to be accessible outside the class. Note that the keyword public allows other classes to access the method from outside the class. The keyword private can be used to restrict access to the method to the class where it is defined. Thus, an Accessor
How to call a private method
In Java, all methods are public by default. You can restrict access to a method by using the keyword private or protected. The keyword private makes the method accessible only to the class it is defined in. The keyword protected allows access to the class or subclass. The protected keyword allows the method to be accessed from within the class and any subclasses.
How to call a private method in java from another class without instantiating object?
Since the method is private, you can call it from any other class. However, to call a private method in java without instantiating an object, you must first declare it static as static methods can be called without an instance of the class.