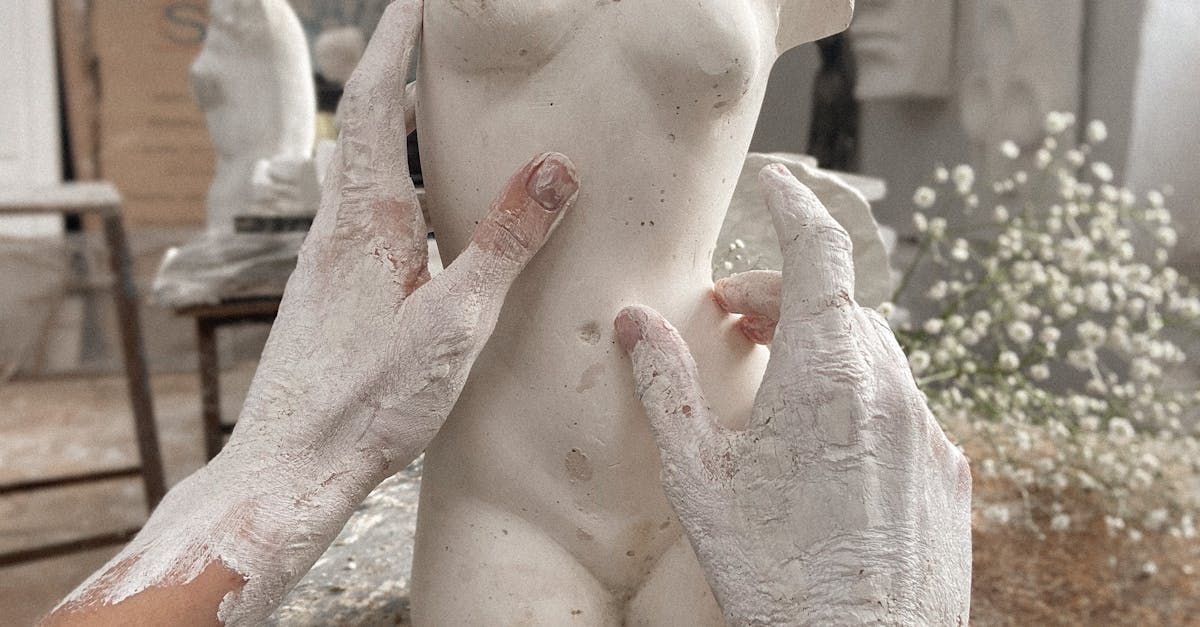
How to create arrays in c?
Similar to C++, the C language also allows you to create an array using the C ‘{’ braces ‘}’ symbol. This is called a ‘nested’ array. A nested array in C consists of a number of elements that are all of the same type. You can use the ‘=’ sign to declare your array of variables. An example of a nested array in C is shown in the following code:
How to create an array of objects in c?
arrays of objects can be created by using the structure definition or the pointer declaration. The structure contains the name of the variable, the data type of each item, and the number of elements within the array. For example, to create an array of 10 integers, you can use the following structure definition:
How to create an array of pointers in c?
Now, the question that follows is how to create an array of pointers in C. When you declare an array, the size of the array is known at compile time. You can declare an array of pointers by using square brackets in front of the name of the variable, but you need to declare a pointer first. In a single line, you declare an array of pointers with the following syntax:
How to create two arrays in c?
To create two arrays in C with their initial size, we use the following syntax: int a[10]; This creates an array of 10 int elements. Note that, we don’t use a length to initialize the array size. The array size is based on the number of elements that are automatically assigned to it. To create two arrays, we can use int a[10], b[20]; This will create two arrays, a with 10 elements and b with 20 elements.
How to create an array of a struct in c?
An array of a struct can be created using the malloc function. It takes the number of elements you want to create in the array as the first argument and the size of each element of the array as the second argument. You have to make sure to include the size of the data member you want to use inside the structure when allocating memory to the array.